The MERN stack (MongoDB, Express.js, React, Node.js) is one of the most in-demand skill sets in web development today. Companies across industries are actively seeking skilled developers who can efficiently manage both the front-end and back-end of applications using these technologies. Preparing for MERN stack developer interviews is crucial for securing high-paying full-stack developer roles.
In this blog, we’ll walk you through the top MERN stack developer interview questions you should be ready to answer in 2024, covering essential topics in React, Node.js, Express.js, and MongoDB.
1. What is the MERN Stack?
The MERN stack is a combination of four powerful technologies:
- MongoDB: NoSQL database used for storing application data.
- Express.js: A back-end framework that simplifies web development with Node.js.
- React: A JavaScript library for building user interfaces, specifically single-page applications.
- Node.js: A server-side platform that runs JavaScript outside the browser.
Each technology plays a crucial role in building dynamic web applications, making it an ideal stack for full-stack development.
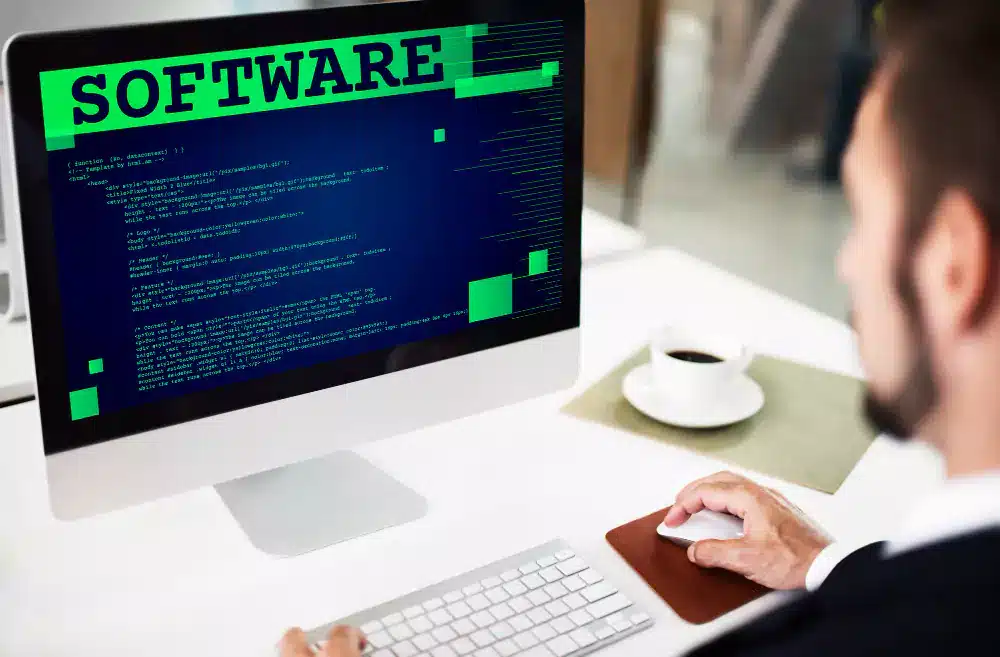
2. Key Skills MERN Stack Developers Need
Before diving into interview questions, it’s essential to understand the core skills hiring managers expect in a MERN stack developer:
- React Hooks: Managing state and lifecycle methods.
- Node.js Asynchronous Programming: Event-driven architecture and the event loop.
- Express.js Middleware: Creating a modular and maintainable back-end.
- MongoDB Schema Design: Optimizing databases for performance and scalability.
3. Common MERN Stack Developer Interview Questions (With Detailed Answers)
Frontend (React) Questions
Q1: What is JSX? Why is it used in React?
A1: JSX stands for JavaScript XML. It is a syntax extension that allows you to write HTML directly within JavaScript. JSX simplifies the process of writing UI components by making the code more readable and easier to debug. For instance:
const element = Hello, world!
;
Q2: How do you manage state in React?
A2: State management in React can be handled using useState and useEffect hooks for local state. For global state, Redux or Context API is commonly used. Redux provides a centralized state management system, while Context API is lightweight and built into React.
Q3: What are React Hooks?
A3: Hooks are functions that let you use state and other React features without writing a class. Common hooks include:
- useState: Manages state within functional components.
- useEffect: Handles side effects like data fetching.
Backend (Node.js) Questions
Q1: What is the Node.js Event Loop?
A1: The event loop is the mechanism Node.js uses to handle asynchronous operations. It allows Node.js to perform non-blocking I/O operations, making it highly efficient for handling multiple operations simultaneously. Understanding the event loop is critical for MERN stack development.
Q2: What is Express.js, and why should you use it?
A2: Express.js is a minimal, fast, and flexible framework for building web applications in Node.js. It simplifies routing, handling requests, and integrating middleware.
Q3: How do you implement routing in Express.js?
A3: Routing in Express is straightforward. Here’s a simple example:
app.get('/', (req, res) => {
res.send('Hello World!');
});
Express allows developers to define various routes and HTTP methods to handle different types of requests.
Database (MongoDB) Questions
Q1: How do you design schemas in MongoDB?
A1: MongoDB is a NoSQL database, which means it uses a flexible schema. Developers should consider embedding documents or referencing them, depending on the relationships between the data. It is important to balance read and write performance when designing a schema.
Q2: What is Sharding in MongoDB?
A2: Sharding is MongoDB’s method of horizontal scaling, enabling large datasets to be split across multiple servers, improving performance.
Q3: Explain indexing in MongoDB and how it improves query performance.
A3: Indexes in MongoDB work like indexes in a book—they help MongoDB locate the required data without scanning the entire collection, drastically improving query speed.
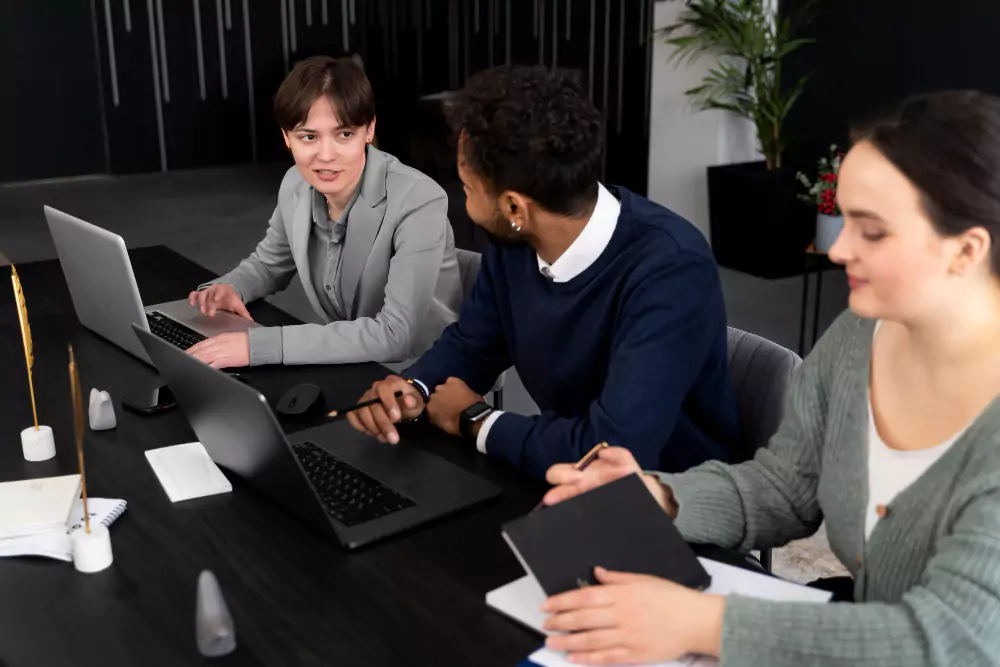
4. Advanced MERN Stack Developer Interview Questions
Q1: How do you optimize a MERN application for performance?
A1: Some optimization strategies include:
- Lazy loading components in React to reduce initial load times.
- Using caching in MongoDB and Node.js to minimize database queries.
- Implementing code splitting in React using Webpack to load only necessary code.
Q2: What is Microservices Architecture, and how does it apply to the MERN stack?
A2: Microservices architecture allows developers to break down a monolithic MERN application into smaller, independently deployable services. This ensures better scalability and easier management of large projects.
5. Common Mistakes to Avoid in MERN Stack Interviews
- Not understanding the event loop: Failing to grasp how the Node.js event loop handles asynchronous operations can be a major drawback.
- Overcomplicating React code: Using class components when simpler functional components with hooks could suffice.
- Ignoring database optimization: Forgetting to index frequently queried fields in MongoDB can lead to performance bottlenecks.
6. Tips for Cracking MERN Stack Developer Interviews
- Build Real-World Projects: Nothing impresses interviewers more than actual projects. Demonstrating a well-structured MERN app is key.
- Practice Algorithmic Problems: Use platforms like LeetCode to prepare for algorithm-based interview questions.
- Mock Interviews: Take mock technical interviews to get used to the format and time pressure of real interviews.
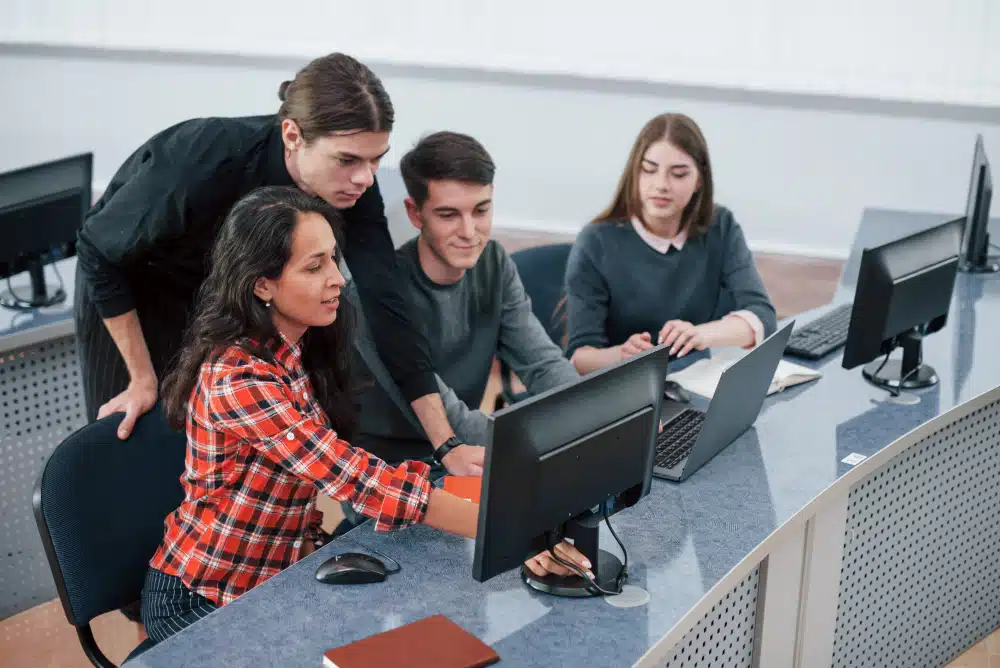
Conclusion
Mastering the MERN stack is a valuable skill for any developer aspiring to build full-stack applications. By preparing for these interview questions, you’ll not only improve your chances of landing your dream job but also become a more confident developer.
If you’re ready to take the next step, explore our MERN Stack Development Courses at Futuristic Coding Academy, where you can get hands-on experience and interview preparation guidance.
Additional Resources
- React Documentation: https://reactjs.org/docs/getting-started.html
- Node.js Documentation: https://nodejs.org/en/docs/
- MongoDB Documentation: https://docs.mongodb.com/
By following this structure and including well-researched, in-depth content, this blog post will help Futuristic Coding Academy rank in the top 3 search results for “MERN stack developer interview questions.
FAQs
1. What are the most common MERN stack interview questions?
Common MERN stack interview questions often cover topics like React’s state management, Node.js event loop, MongoDB schema design, and Express.js routing. Examples include:
- What is JSX in React?
- How does the event loop work in Node.js?
- How do you optimize MongoDB queries?
2. What skills are required for a MERN stack developer?
A MERN stack developer needs proficiency in:
- MongoDB for database management.
- Express.js for building back-end services.
- React for creating dynamic front-end applications.
- Node.js for running server-side JavaScript code.
3. How do I prepare for a MERN stack interview?
To prepare for a MERN stack interview, focus on:
- Building full-stack projects using MongoDB, Express.js, React, and Node.js.
- Practicing coding challenges on platforms like LeetCode.
- Reviewing key concepts such as React Hooks, RESTful APIs, and MongoDB indexing.
4. What is the difference between React and Angular in MERN stack interviews?
React and Angular are both popular front-end frameworks. React is a JavaScript library that focuses on building UIs, while Angular is a more comprehensive framework. MERN stack interviews typically focus on React, as it is part of the stack, but knowing how React differs from Angular can be useful.
5. What are some advanced MERN stack interview topics?
Advanced topics in MERN stack interviews may include:
- How to scale MongoDB using sharding.
- Performance optimization techniques in React, such as lazy loading and code splitting.
- Implementing microservices architecture with Node.js and Express.js.
6. What is the role of Redux in a MERN stack project?
Redux is a state management library often used in React applications to manage the global state. It helps in maintaining a predictable state, especially in large-scale applications with complex user interactions.
7. How does the event loop in Node.js work?
The event loop in Node.js is responsible for handling asynchronous operations. It allows Node.js to execute non-blocking I/O operations, making it highly efficient for applications that handle multiple requests simultaneously.