In today’s digital age, full-stack development skills are in high demand, and the MERN stack is one of the most sought-after. If you’re looking to develop modern web applications and master the full stack, this guide is your go-to resource. Here, we break down each part of the MERN stack—MongoDB, Express, React, and Node.js—and guide you through the journey of building robust web applications from scratch.
What is the MERN Stack?
The MERN stack is a combination of four powerful technologies:
- MongoDB: A NoSQL database for storing data as JSON documents.
- Express.js: A lightweight backend framework for handling web requests.
- React.js: A front-end library for building interactive UIs.
- Node.js: A JavaScript runtime that allows you to build the server side of your application.
Together, these technologies offer developers a cohesive stack for building full-stack JavaScript applications where you can write both frontend and backend in JavaScript. This uniformity speeds up development and provides a seamless workflow.
Why Learn the MERN Stack?
1. Single Language for Full-Stack Development
One of the biggest advantages of MERN is that it uses JavaScript throughout the stack. You don’t have to learn multiple programming languages for frontend and backend development—JavaScript powers everything!
2. High Demand and Lucrative Salaries
MERN developers are in high demand because companies today are focused on building dynamic, real-time applications. This skill set not only helps you secure high-paying jobs but also offers freelance opportunities.
3. Open-Source and Free to Learn
All components of the MERN stack are open-source, making it accessible for anyone to learn and develop.
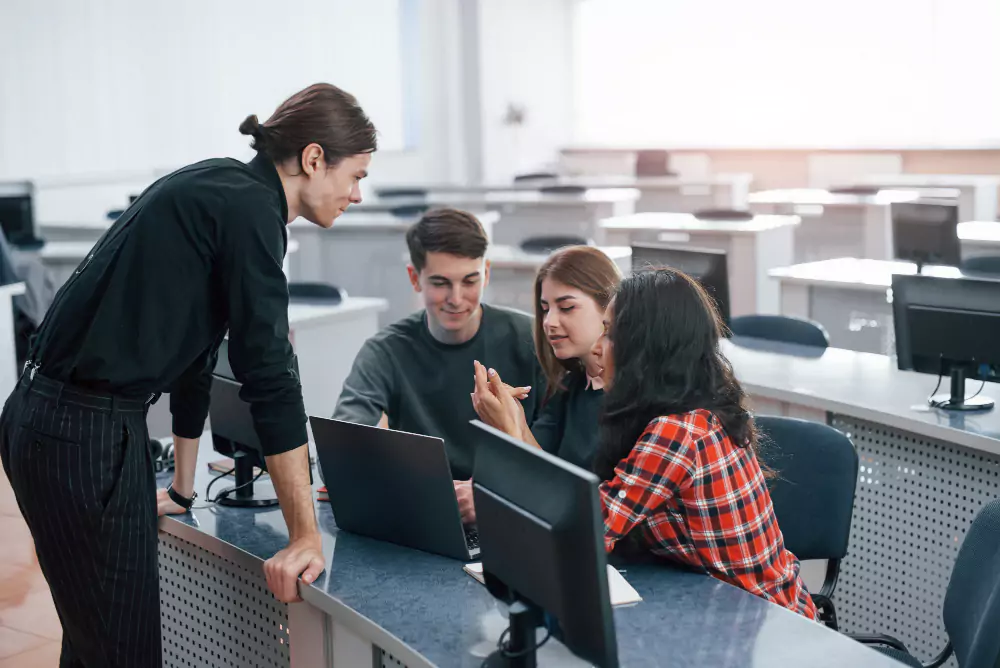
Prerequisites for Learning the MERN Stack
Before diving into the MERN stack, it’s recommended that you have a basic understanding of JavaScript as well as familiarity with HTML, CSS, and Git. Having a working knowledge of API architecture and basic programming concepts (such as variables, loops, and functions) will also make your learning journey smoother.
Step-by-Step Guide: Building a MERN Stack Application
1. Setting Up the Development Environment
Start by installing the essential tools:
- Node.js and npm (Node Package Manager)
- MongoDB (set it up locally or use a cloud service like MongoDB Atlas)
- React via Create React App for frontend setup
npm install create-react-app
2. Backend: Setting Up Express.js and Node.js
Initialize a Node.js project by creating a new directory and running:
npm init -y
npm install express mongoose dotenv
Create your Express.js server:
const express = require('express');
const app = express();
app.use(express.json());
app.listen(5000, () => {
console.log("Server is running on port 5000");
});
3. Database: Connecting MongoDB with Mongoose
Use Mongoose to create a schema and interact with the MongoDB database:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mernDB', {
useNewUrlParser: true,
useUnifiedTopology: true
});
4. Frontend: Building UI with React
In your React app, use React Hooks and state management to handle user input and display data from your backend. Here’s a sample component:
import React, { useState } from 'react';
function App() {
const [data, setData] = useState(null);
const fetchData = () => {
fetch('/api/data')
.then(response => response.json())
.then(data => setData(data));
};
return (
{data}
);
}
export default App;
5. Full Integration: Connecting Frontend to Backend
Use Axios to send HTTP requests from React to your Express backend.
axios.get('/api/data').then(response => setData(response.data));
On the backend, define the API route:
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello, MERN!' });
});
6. Authentication: Securing Your MERN Application
Implement JWT (JSON Web Tokens) for secure authentication:
On the backend, create JWT tokens on user login:
const jwt = require('jsonwebtoken');
const token = jwt.sign({ id: user._id }, 'your-secret-key');
On the backend, create JWT tokens on user login:
localStorage.setItem('token', token);
axios.get('/api/protected', { headers: { Authorization: `Bearer ${token}` } });
7. Deploying Your MERN Application
Deploy your full-stack MERN application to Heroku or AWS:
Push your code to GitHub.
Set up a Heroku application:
heroku create
git push heroku main
Advanced Concepts: Beyond Basics
Once you’re comfortable with the basics of MERN, dive deeper into advanced topics:
- WebSockets for real-time communication (useful for chat applications).
- GraphQL as an alternative to REST APIs.
- Microservices architecture for scaling large applications.
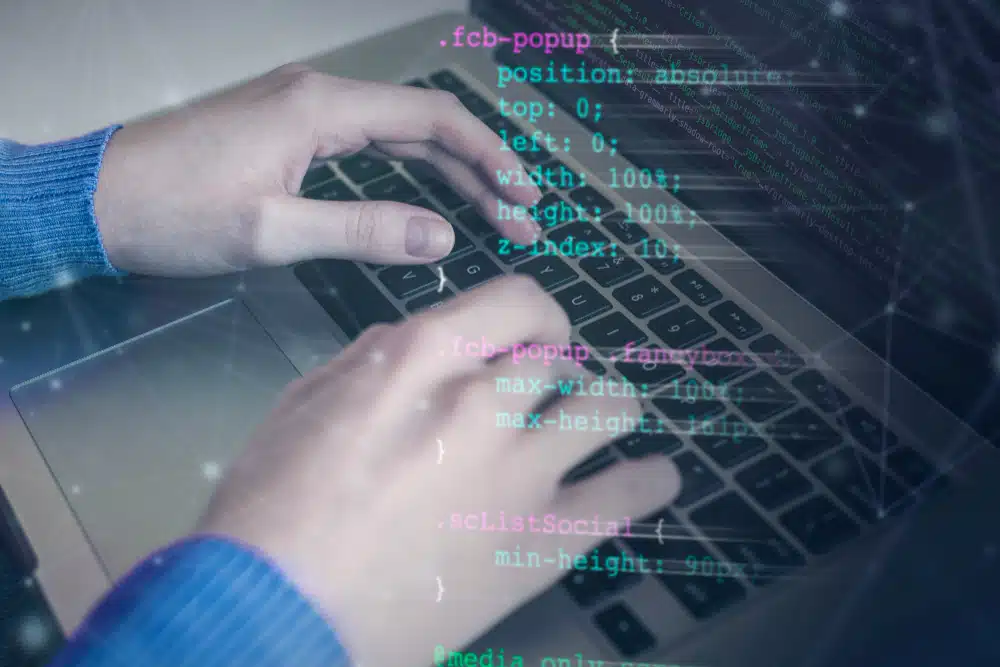
Common Issues and Troubleshooting in MERN Development
Here are some typical issues you might encounter:
- CORS errors: Fix by using the
cors
package in Express. - Database connection errors: Check MongoDB connection strings or network configurations.
- Deployment issues:
Ensure environment variables are properly set in platforms like Heroku or AWS.
Conclusion: Take Your First Step
Ready to become a full-stack developer? Start by building a simple CRUD app, and then gradually add complexity—user authentication, real-time features, and cloud deployment. With the MERN stack, the possibilities are endless!
FAQs
What are the prerequisites for learning MERN Stack?
Basic JavaScript, HTML, CSS knowledge, and familiarity with Git and databases.
How long does it take to learn the MERN Stack?
Depending on your experience, it could take anywhere from a few weeks to a few months.
What projects can I build with the MERN stack?
From simple portfolio websites to complex eCommerce platforms, you can build almost anything!